Welcome to another issue!
Hey! π
In the upcoming weeks, I won't have any newsletter sponsors. If you have a tool, an article, a new project, or something else you'd like to share with 20K developers, please reply to this newsletter.
With the next issue, I will help one person reach more developers. π
I appreciate your support and let's dive into this week's news! π

Subscribe to another newsletter I run!
3750 subscribers already! π
Weekly newsletter featuring the best tools for iOS developers. Subscribe if you like @iOS developers
Swift Around the Web
What's new in Swift 6.0?
Swift 6.0 brings a significant update to the Swift programming language, with a strong focus on improving concurrency safety.
Here's a breakdown of the key changes:
- Safer Concurrency by Default: Enforces writing code that avoids data races.
- Enhanced Concurrency Features: Isolation regions and the sending keyword provide better tools for developers to write safe and efficient concurrent code.
New Language Features:
- count(where:) for filtering and counting elements in collections (SE-0220).
- Typed throws, to specify the types of errors a function can throw (SE-0413).
- Support for non-copyable types in generics and protocols (SE-0427, SE-0432). 128-bit integer types for wider numeric ranges (SE-0425).
Introduction to Swift Service Lifecycle
Swift Service Lifecycle, a library for simplified application startup and shutdown with freeing resources before exiting.
Core Concepts:
- Define services (long-running tasks) with Service protocol.
- Manage multiple services concurrently with ServiceGroup.
- Gracefully shut down services using gracefulShutdown.
It provides efficient resource management through graceful shutdowns, reusable shutdown logic across applications, and simplified concurrent task management.
Designing a Declarative API
A custom validator type in Swift for user input validation. It leverages declarative programming for clear logic and structure, reducing control flow concerns. Notably, this approach is applicable beyond UI development.
Key Concepts:
- Define validation rules using predicates (functions checking validity).
- Chain rule methods for a declarative and DSL-like style.
- Combine validators with custom operators (AND, OR) for complex logic.
This article demonstrates how to design a declarative API using a validator example, promoting code readability, maintainability, and flexibility.
Coding
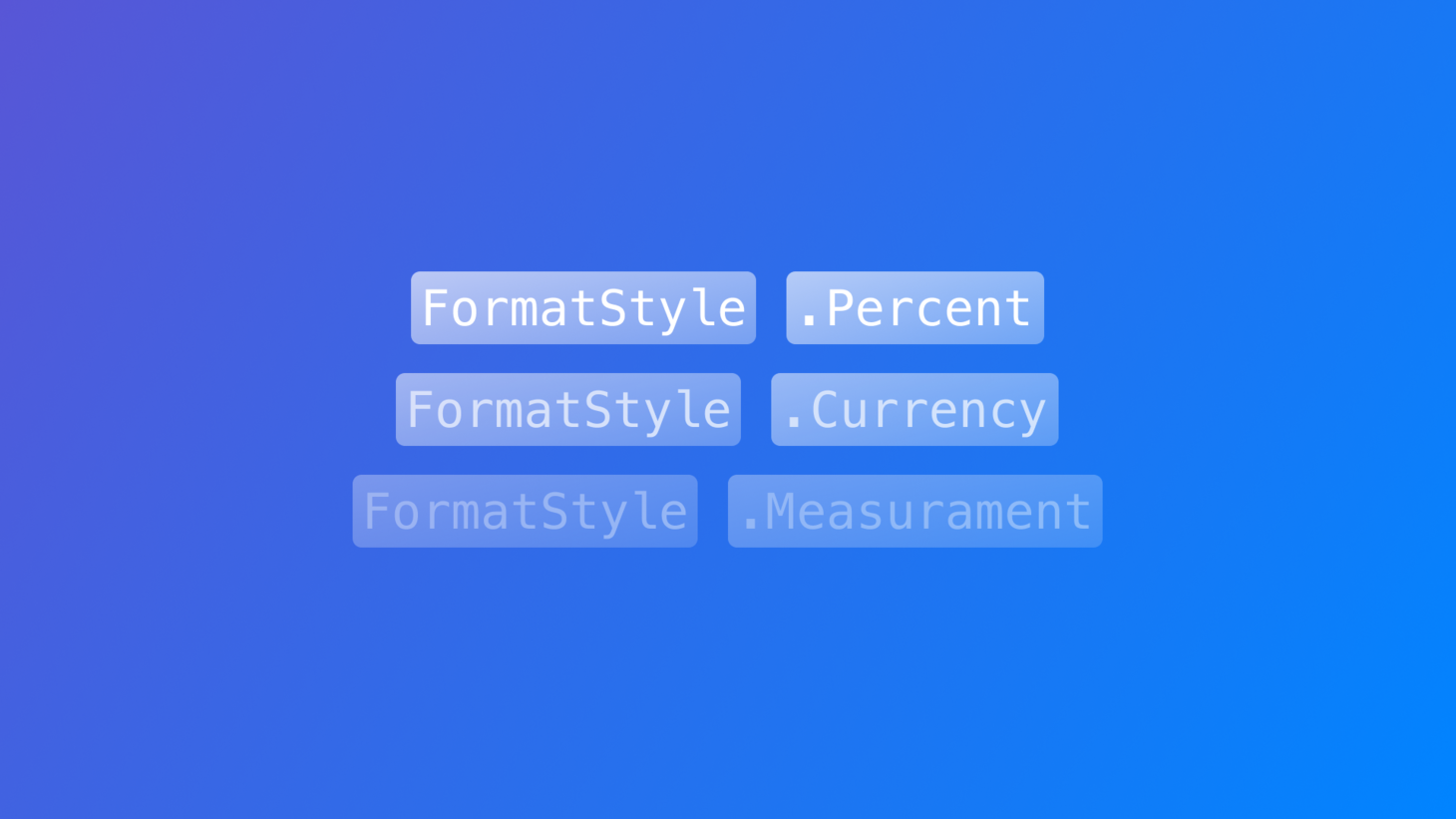
Formatting data as text in a Text view in SwiftUI
SwiftUI's Text view offers various formatting options for displaying data like currency, dates, measurements, etc.
Key formatters:
- Decimal.FormatStyle: Currency, percentages.
- Measurement.FormatStyle: Units and measures.
- Date.FormatStyle: Dates and times.
- ListFormatStyle: Lists with commas and conjunctions.
- PersonNameComponents.FormatStyle: Person names.
Scene types in a SwiftUI Mac app
Explore various scene types available in SwiftUI for building macOS applications. That is crucial for creating versatile and efficient apps.
Key Scene Types:
- WindowGroup, DocumentGroup: Manage multiple windows or documents, with macOS features like tabbed windows and document handling.
- Settings: Creates a dedicated window for app preferences.
- Window: Creates a single, unique window for tools or utilities.
- MenuBarExtra: Adds a persistent control to the menu bar for quick access to core features.
visionOS
Introduction to RealityView
RealityView is a powerful SwiftUI view for 3D models and effects in VisionOS apps. It offers more control over 3D content compared to Model3D.
Core Functionality:
- Entities: Building blocks for a scene, representing visible/invisible objects.
- Interactive Experiences: Update entities based on app state.
- Components: Enhance entities with functionalities (gestures, collision, etc.).
- RealityKit Integration: Leverages RealityKit for features like simulation and AR.
Overall, RealityView unlocks the possibilities for creating interactive and engaging 3D experiences in your VisionOS apps.
Apple News
WWDC 24 Keynotes
Appleβs Worldwide Developers Conference 2024 keynote has come to a close β and the company has a lot to share.
- Apple Intelligence: New on-device AI for image creation, and text summaries.
- Siri Upgrade: Deeper iPhone integration, leverages ChatGPT, and improves understanding.
- iOS 18: AI enhances emails, photos, and the Control Center allowing app lock.
- Apple TV+ & Passwords app: Streamlined access to info, and login management across devices.
- Focus on iPad & Apple Watch: New features for productivity and health.
- VisionOS 2 & AirPods Pro: Enhanced displays, workouts, and Siri control.
Design
iOS 18: Notable UIKit Additions
This blog post highlights new features in iOS 18's UIKit framework for building user interfaces.
Here's a quick rundown:
- Automatic trait tracking: Less code for handling UI changes (light/dark mode, etc.).
- UIUpdateLink: New animation tool for smoother performance.
- Enhanced SF Symbols: New animations (wiggle, breathe, rotate) and more animation control.
- Rich text editing: Developers can offer features like bold and italics in their apps similar to notes.
- More features: Week-by-week date selection, unified gestures across frameworks, haptic feedback for drawing events, system-invoked commands on iPhone, and improved sidebars/tab bars.
Other Cool Stuff
ChatGPT for Swift: Top 5 code generation prompts
Explore how to leverage ChatGPT, an AI chatbot, to boost your productivity when writing Swift code.
Creating gradient on polylines in SwiftUI MapKit
This post offers a solution for creating polylines with gradients in SwiftUI using MapKit. Standard linearGradient struggles with desired gradient placement on the map.
We can use the stroke
modifier with a Gradient object directly to achieve gradient polylines on the map.
How to create custom @Environment values in SwiftUI
Create custom @Environment values to share data across views without passing it explicitly.
Key Steps:
- Define an EnvironmentKey type (e.g., ThemeEnvironmentKey).
- Extend EnvironmentValues with a property for the key (e.g., .theme).
- Use @Environment to inject the value into views (e.g., access .theme in LoginView).
It helps to write cleaner code by avoiding explicit data passing between views and centralized management of shared data.
Videos
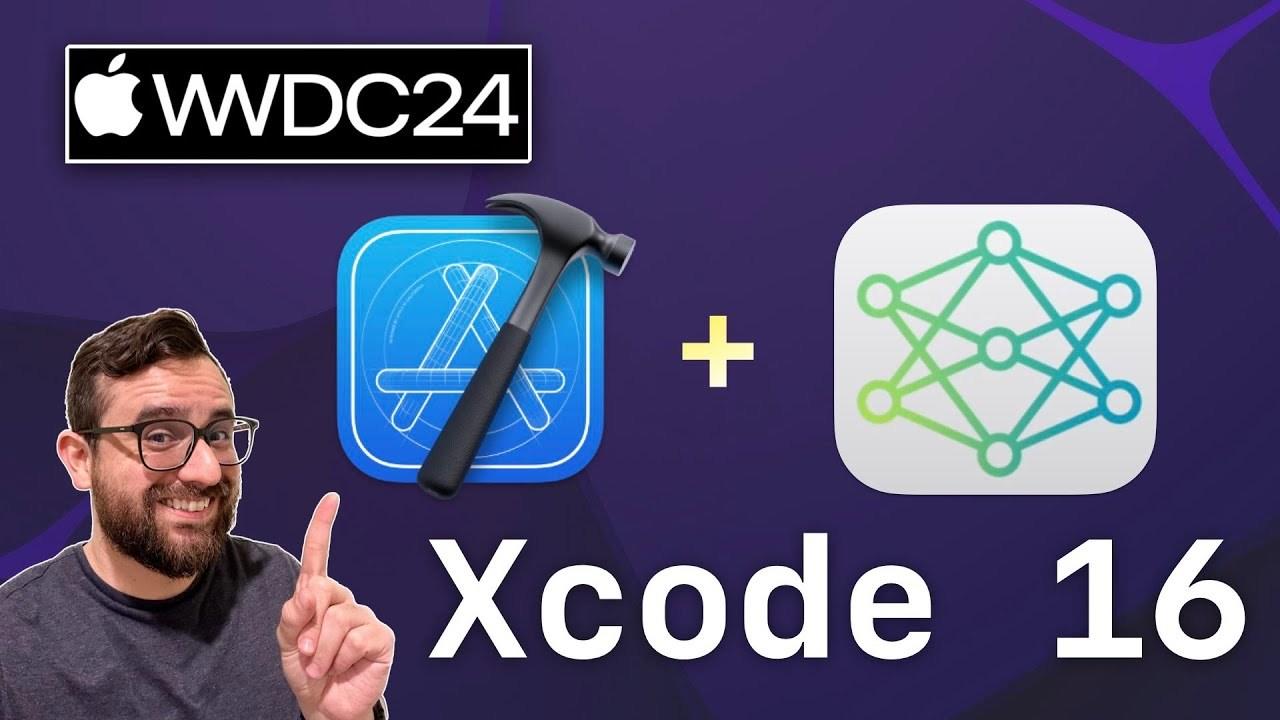
First Look at Xcode 16's Code Completion
The new features of Xcode 16 beta, include an AI-powered code completion tool called Apple Intelligence.
What's new?
- Xcode 16 beta introduces code completion powered by Apple Intelligence.
- As you type, Apple Intelligence suggests code snippets, function names, and variable names.
Limitations?
- The video shows instances where the AI struggles with complex tasks or user intent.
- The reviewer suggests that AI needs further development for better accuracy.