Welcome to another issue!
Subscribe to another newsletter I run!
3650 subscribers already! 🚀
Weekly newsletter featuring the best tools for iOS developers. Subscribe if you like @iOS developers
Sponsor
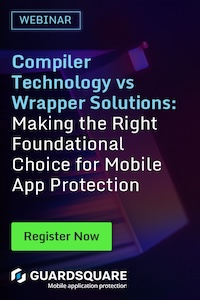
Compiler Technology vs Wrapper Solutions: Making the right foundational choice for mobile app protection (Webinar)
The right mobile app protection secures apps and SDKs against threats such as reverse engineering, back-end abuse, app cloning, piracy, and IP theft, to name a few. Join our webinar for a nuanced explanation of mobile app protection, comparing compiler-based technology and wrapper solutions.
Swift Around the Web
Build your next website in Swift
Exploring the idea of using Swift, the programming language behind iPhone apps, to build websites. While not a complete replacement, it offers potential advantages:
- Fewer Errors: Swift's built-in checks can prevent website bugs.
- Familiar for Developers: Programmers can use their existing Swift knowledge.
However, current methods lack features for responsiveness (adapting to different screens) and accessibility (catering to all users).
Difference Between try, try? and try! and When to Use Them in Swift
Learn to handle errors using try, try?, and try! in Swift.
- try: Used for functions that might throw errors. You need to handle the error with do-catch blocks or propagate it.
- try?: Attempts a throwing function. If an error occurs, the result becomes nil. Useful when you don't need to handle the specific error.
- try! (or else!): Force unwraps the result of a throwing function. If an error occurs, your app crashes. Use with caution, only when you're sure the function won't throw.
Developing Embedded Applications with Swift
Using Swift for embedded development on the SwiftIO platform gives the advantages of high-level languages like Swift for complex embedded applications and explores the SwiftIO Playground Kit as a development environment.
- Challenges: Limited development environment and slow data transfer times exist.
- Solution: The author creates simulated controls to test programs in a familiar Swift environment, enabling reusable code for both embedded systems and regular computers.
This approach simplifies development and testing across platforms.
Code
What Does spacing = nil Mean in SwiftUI?
Explores the concept of spacing in SwiftUI, particularly the default value nil for the spacing parameter in layout containers.
- By default (spacing = nil), SwiftUI calculates space between elements based on their type, device, etc. This spacing can be inconsistent.
- You can set a custom spacing value to control the space between elements uniformly.
- Even negative spacing values are allowed for specific layouts.
- In some cases, spacing isn't used to add gaps but for layout adjustments.
MVVM: An architectural coding pattern to structure SwiftUI Views
MVVM (Model-View-ViewModel), a popular architectural coding pattern for structuring SwiftUI applications. It separates the concerns of views (presentation), view models (data and logic), and models (data structures).
- Enhanced Reusability: Views become independent of specific data models, allowing them to work with various view models.
- Improved Testability: Business logic resides within isolated view models, simplifying unit testing.
- Clearer Code Structure: Separation of concerns leads to well-organized and easier-to-understand code.
Design
How To Generate Complex Shape Paths From SF Symbols For Path Animations
SwiftUI offers a simpler method for animating complex shapes using SF Symbols. Animating intricate shapes with curves in SwiftUI can be difficult due to complex control point calculations.
How to create:
- Extract SF Symbol as SVG: Access individual shape properties for animation.
- Edit SVG (Optional): Modify in design tools (e.g., Figma) for customization.
- Convert SVG to Path & Animate: Use online tools to convert and animate using trim(from:to:).
Random Cool Stuff
SwiftUI Previews and Preview Content Are Excluded From App Store Builds
This article warns about unexpected behavior in Xcode regarding SwiftUI previews and App Store builds.
- The Issue: Preview code and related assets are included in unoptimized builds, potentially causing problems during archiving (preparing for App Store submission).
- The Fix (for now): Wrap both SwiftUI previews and development assets in #if DEBUG statements. This ensures clean builds and prevents leaks of test data into the final app.
Cleaner builds and avoiding unintended leaks are crucial for a smooth App Store submission process.
On Fluent Models and Sendable warnings
Fluent Models addresses a warning in FluentKit 1.48.0 related to model types.
- The Problem: Models trigger "Sendable" warnings due to Fluent's property wrappers.
- The Fix: Suppress the warning with @unchecked Sendable on your model types.
- Why This Happened: Technical limitations with reference types and mutability in Fluent models clash with Swift's "Sendable" requirement.
- The Future: This is a temporary solution. A proper fix is promised in Fluent 5.
In Case You Missed It
Discovering app features with TipKit. Rules
Learn how to leverage TipKit's "rules" to control when feature tips appear in your app. This ensures users see relevant tips at the right time, enhancing the onboarding experience.
Key functionalities of "rules":
- Define conditions for tip display (e.g., "pro user" tip).
- Link tips to app state and user actions.
- Combine rules for complex logic.
- Control tip frequency with options.
Videos
Valuable Swift and SwiftUI techniques
The Deep Dish Swift 2024 conference, showcases various productivity hacks for iOS developers. While the presenter couldn't attend due to COVID, they've shared a recording of an early rehearsal.
Key takeaways from Stewart Lynch:
- Effortless Layouts: Master frame alignments for precise UI.
- Dynamic UI Elements: Create custom views that adapt to content size.
- Advanced Text Tools: Leverage built-in features for text handling.
- Streamlined UI Testing: Generate preview screenshots for efficient testing.
Did you know that Xcode Previews also work with UIKit?
Xcode 15 introduces a new and simpler way to use Xcode Previews in a UIKit-based project using the #Preview macro. This macro supports a wider range of views compared to previous versions:
- SwiftUI views
- UIKit views (UIView, UIViewController)
- AppKit views (NSView, NSViewController)